Python Programming Training
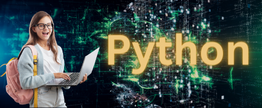
Məhsul təsviri
Overview
A Python programming training overview typically covers a range of topics, tailored to the needs and proficiency levels of the participants. Here's a general outline of what might be included in such a training program:
1. **Introduction to Python:**
- Overview of Python and its features.
- Installation and setup of Python environment.
- Running Python code using interactive interpreter and scripts.
2. **Basic Syntax and Data Types:**
- Variables and data types (integers, floats, strings, lists, tuples, dictionaries, etc.).
- Basic operators and expressions.
- Control flow structures (if statements, loops, etc.).
3. **Functions and Modules:**
- Defining and calling functions.
- Parameters and return values.
- Scope of variables.
- Importing modules and using built-in functions.
4. **Data Structures:**
- Lists: indexing, slicing, list methods.
- Tuples: creation, unpacking.
- Dictionaries: accessing, modifying, iterating.
- Sets: creation, methods.
5. **File Handling:**
- Reading from and writing to files.
- Working with different file formats (text files, CSV, JSON, etc.).
6. **Exception Handling:**
- Understanding exceptions and error handling.
- Try-except blocks.
- Handling specific exceptions.
7. **Object-Oriented Programming (OOP):**
- Introduction to OOP concepts (classes, objects, inheritance, polymorphism, encapsulation).
- Creating classes and objects.
- Class methods, instance methods, and static methods.
- Special methods (e.g., `__init__`, `__str__`).
8. **Advanced Topics (Optional, Depending on the Audience's Needs):**
- Regular expressions.
- Decorators.
- Generators and iterators.
- Multithreading and multiprocessing.
- Database access with Python (e.g., using SQLite or ORM like SQLAlchemy).
9. **Introduction to Libraries and Frameworks:**
- Overview of popular libraries and frameworks (e.g., NumPy, Pandas, Matplotlib, Flask, Django).
- Basic usage examples and where they might be applicable.
10. **Best Practices and Code Quality:**
- Writing clean and readable code.
- Code commenting and documentation.
- Code optimization and efficiency.
- Version control with Git.
11. **Practical Projects and Exercises:**
- Hands-on coding exercises to reinforce learning.
- Small projects to apply concepts learned during the training.
12. **Advanced Applications (Depending on the Focus of the Training):**
- Web scraping.
- Data analysis and visualization.
- Web development.
- Machine learning and data science.
This outline can be customized based on the level of expertise of the participants, the duration of the training, and specific learning objectives. Additionally, hands-on practice, real-world examples, and interactive sessions should be integrated throughout the training to enhance learning and retention.